1 min to read
Python in IoT and Robotics
Backend Solutions for Connected Systems
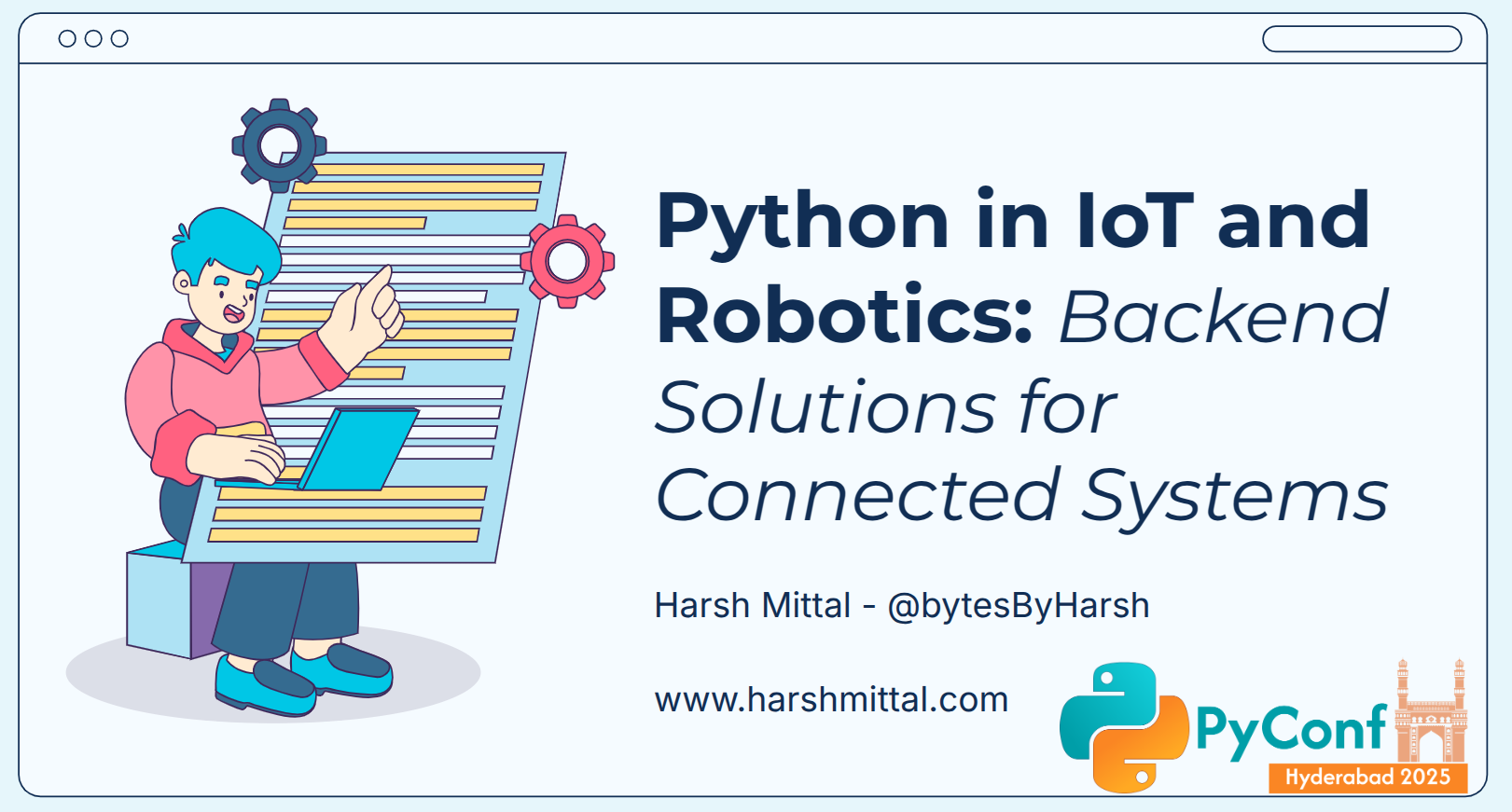
Python in IoT and Robotics
Conference Talk Link: https://2025.pyconfhyd.org/speakers/harsh-mittal
Github Link: https://github.com/bytesByHarsh/PyConfHyd2025
More content will be updated after the talk!!!
Introduction
Imagine a world where your toaster chats with your coffee machine about the perfect brew temperature, or where a fleet of drones gossip about their battery levels and GPS coordinates as they fly around. Welcome to the realm of IoT and robotics, where devices are not only smart—they’re downright social! In my recent talk, “Python in IoT & Robotics: Scalable Backend Solutions for Connected Systems,” I dove into how Python serves as the central nervous system for orchestrating these connected systems. Here’s a deeper look at the ideas and architectures we explored.
The Need for a Connected Backend
Breaking Down the Silos
In many robotics projects, devices operate in isolation, much like a teenager who refuses to share their smartphone. These “data silos” make it difficult to harness the full potential of IoT systems. Our first challenge? Getting these smart devices to talk to each other.
Comments